Subway Map Visualization jQuery Plugin
I have always been fascinated by the visual clarity of the London Underground map. Given the number of cities that have adopted this mapping approach for their own subway systems, clearly this is a popular opinion. At a conference some years back, I saw a poster for the Yahoo! Developer Services. They had taken the concept of a subway map and applied it to create a YDN Metro Map. Once again, I was in awe of the visual clarity of this map in helping one understand the various Yahoo! services and how they inter-related with each other. I thought it would be awesome if there were a pseudo-programmatic way in which to render such maps to convey real-world ecosystems. A few examples I can think of:
- University departments, offices, student groups
- Government
- Open Source projects
- Internet startups by category
More examples on this blog: Ten Examples of the Subway Map Metaphor.
Fast-forward to now. Finally, with the advent of HTML5 <canvas> element and jQuery, I felt it was now possible to implement this in a way that with a little bit of effort, anyone who knows HTML can easily create a subway map. I felt a jQuery plugin was the way to go as I had never created one before and also it seemed like the most well-suited for the task. My goals:
- Anyone should be able to create a beautiful, interactive subway map visualization for their website using HTML markup
- The map should be as faithful as possible to the London Underground map style with smooth curves and interchange connectors and 45-degree diagonals
- The map size, line width and colors should all be customizable
- Stations, interchanges and linked interchanges should be distinguishable from each other
- The markup used to create the map should be search engine friendly
With these goals in mind, I started creating my jQuery plugin. A few days of concentrated effort later, I had a working subwayMap plugin and am quite pleased with the result. You can download the plugin below and documentation follows in this post. I hope you will give the plugin a try and find it useful.
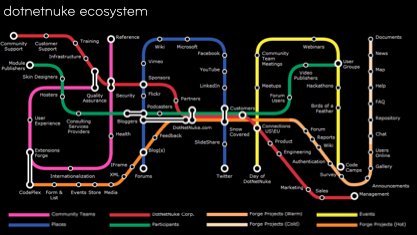
Download
[download id=”11″] or get it on GitHub
Step-by-Step Guide
Here is a guide to using the Subway Map Visualization jQuery Plugin. Before you get started, there’s one thing you’ll want to keep in mind — beautiful subway maps are never automatic; they are almost always the result of care in design and placement to ensure that the resulting map is functional, legible and beautiful. This plugin is just a tool…you will still need to plan and design your map in order to produce a good result.
Demo: Here’s a demo of the finished map described in this guide.
Referencing the Plugin
The subwayMap plugin is referenced similar to other jQuery plugins by adding a script element to the HTML markup.
<script src="http://code.jquery.com/jquery-1.4.2.min.js" type="text/javascript"></script> <script src="jquery.subwayMap-0.5.0.js" type="text/javascript">
Using the Plugin
The subwayMap plugin is called using a jQuery selector as follows:
$("#sampleselector").subwayMap({ debug: true });
The only supported option (at present) is “debug” which has a default value of “false”. Setting it to true will display some debug statements in the JS console.
HTML Markup for Plugin
Like most navigation plugins, subwayMap uses an unordered list. The basic markup consists of the following:
- An outer DIV element to control general placement, background etc.
- One UL element for each “line” desired in the map.
- For each UL element, one or more LI elements with either plain text or an A element with plain text. An LI element provides coordinates for drawing lines and/or markers on the map.
Each of the DIV, UL and LI elements make use of custom attributes to convey how the map should be rendered. These are explained in the Step-by-Step section below.
Map Rendering
The subwayMap plugin renders the map on a grid with the origin at top left (i.e. X coordinates extend from left to right and Y coordinates extend from top to bottom). The size of this grid depends on a value you define called “cellSize.” For example, if you define a cellSize of 50 and specify a grid of 20 columns by 10 rows, then you will have a map that is 1000 pixels wide and 500 pixels high. For each UL element, a <canvas> element that is the size of the grid is created and positioned at (0,0). Subsequent <canvas> elements are stacked on top of the prior <canvas> elements. Station and interchange markers for each line are also created in separate, stacked <canvas> elements, however their z-Index is always higher than that of the <canvas> elements containing the lines. Finally, all labels are added as elements with the highest z-Index of all the elements in the map.
Creating a SubwayMap Step-by-Step
Now that the basics are out of the way, let’s step through the process of creating a subway map from scratch. I am using jQuery UI as my mapping subject, creating a line for Widgets, Interactions and Effects. Here’s a map and the markup used to create it:
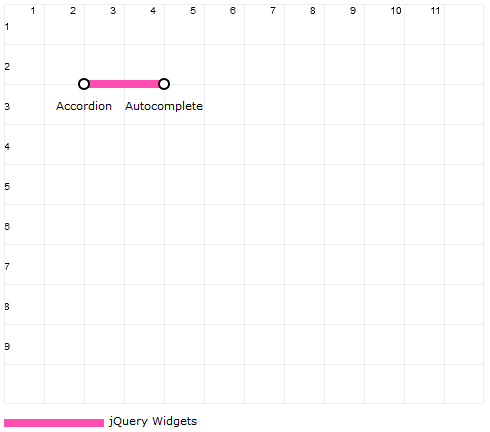
<div data-columns="12" data-rows="10" data-cellSize="40" data-legendId="legend" data-textClass="text" data-gridNumbers="true" data-grid="true" data-lineWidth="8"> <ul data-color="#ff4db2" data-label="jQuery Widgets"> <li data-coords="2,2"><a href="http://jqueryui.com/demos/accordion/">Accordion</a></li> <li data-coords="4,2"><a href="http://jqueryui.com/demos/autocomplete/">Autocomplete</a></li> </ul> </div> <div id="legend"></div> <script type="text/javascript"> $(".subway-map").subwayMap({ debug: true }); </script>
This code looks way more complicated than it actually is. Most of the verbosity comes from the many “data-*” attributes that the plugin uses for all customizations. Here are the attributes being used in the code above:
(DIV) data-columns: The number of columns the map will display (12 in this example)
(DIV) data-rows: The number of rows the map will display (10 in this example)
(DIV) data-cellSize: The width and height of each cell in pixels (40 in this example, resulting in a grid that is 480px wide by 400px tall)
(DIV) data-legendId: The ID of an HTML element into which the map legend will be appended (“legend” in this example)
(DIV) data-textClass: The CSS class to use for text labels in the map (“text” in this example)
(DIV) data-grid: True or false, to show or hide a grid that is useful during map construction. The default is false (“true” in this example)
(DIV) data-gridNumbers: True or false, to show or hide numbers on the grid. Only applies if data-grid=”true” (“true” in this example)
(DIV) data-lineWidth: The width in pixels for each line. The default is 10 pixels. (8 in this example)
(UL) data-color: The color of the line in standard CSS RGB notation (#ff4db2 in this example)
(UL) data-label: The label for the line that will be displayed in the legend (“jQuery Widgets” in this example)
(LI) data-coords: The X,Y coordinate pair where the line should be drawn to from its last location (or the starting location if it’s the first LI element)
As you can see from the illustration, the result of the sample markup was a grid with numbers, a line drawn from (2,2) to (4,2) and finally markers at both coordinate locations. The markers are automatically added whenever you have any content in your LI element and later, we’ll see how you can override the marker type.
Now, let’s extend this line a bit, by adding a few more LI elements. For brevity, I will omit the overall definition and just show the LI elements here:
<li data-coords="2,2"><a href="http://jqueryui.com/demos/accordion/">Accordion</a></li> <li data-coords="4,2"><a href="http://jqueryui.com/demos/autocomplete/">Autocomplete</a></li> <li data-coords="5,4"></li> <li data-coords="7,4"></li> <li data-coords="8,2"></li>
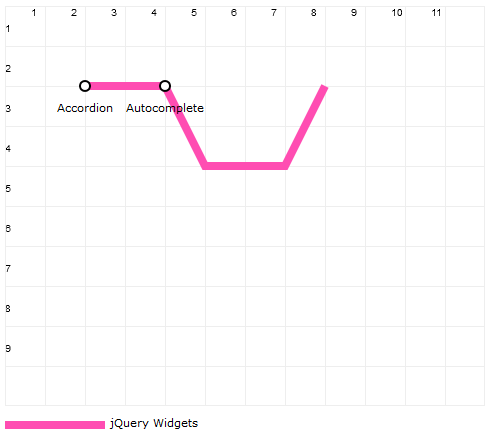
The resulting image is as you would expect. However, it is not very pretty to look at. To make it nicer, we need to add some curves. The plugin provides four directional curves that can be used anytime the difference between both X and Y start and end coordinates is exactly 1. Think of it like plumbing pipes…in order to make a right angle, you introduce an elbow joint. This is somewhat similar. In order to make a smooth, 90-degree curve, you use one cell for the curve as illustrated below:
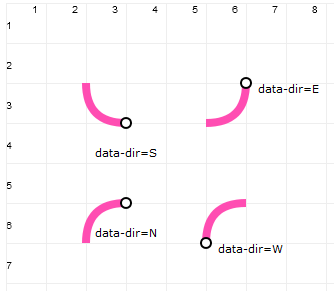
To make a curve, you add a “data-dir” attribute to the LI element that defines the coordinates for the end of the curve. The value of this attribute is directional – E, W, N or S – indicating the direction in which the line will first go before making a right-angle to the coordinate referenced by that LI element. Let’s continue with our example to see this in action:
<li data-coords="2,2"><a href="http://jqueryui.com/demos/accordion/">Accordion</a></li> <li data-coords="4,2"><a href="http://jqueryui.com/demos/autocomplete/">Autocomplete</a></li> <li data-coords="5,3" data-dir="E"></li> <li data-coords="6,4" data-dir="S"></li> <li data-coords="7,4"></li> <li data-coords="8,3" data-dir="E"></li> <li data-coords="8,2"></li>
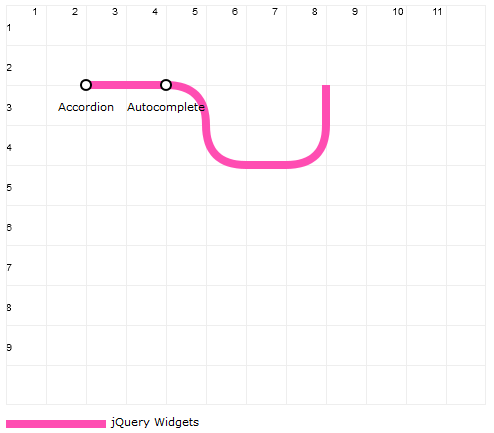
To get the curves, some of the coordinates had to be changed in order to achieve the X and Y coordinate difference of exactly “1”. Note the additional “data-dir” attribute that determines the direction of the curve. The final result is a line that is similar, but not the same as the original and definitely more pleasing to the eye.
Let’s make the line loop around a bit and then go south on a diagonal run.
<li data-coords="2,2"><a href="http://jqueryui.com/demos/accordion/">Accordion</a></li> <li data-coords="4,2"><a href="http://jqueryui.com/demos/autocomplete/">Autocomplete</a></li> <li data-coords="5,3" data-dir="E"></li> <li data-coords="6,4" data-dir="S"></li> <li data-coords="7,4"></li> <li data-coords="8,3" data-dir="E"></li> <li data-coords="8,2"></li>
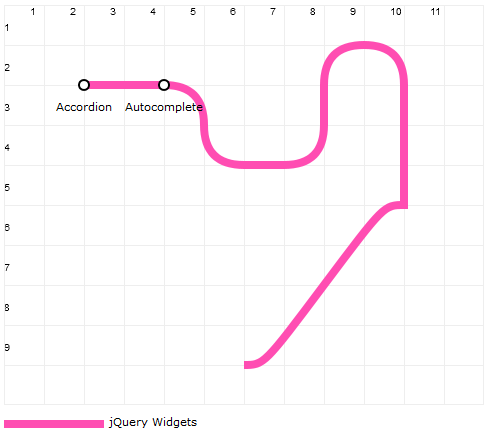
The diagonal line was drawn correctly, but notice the small curves at the beginning and end. These are automatically added when the difference between the X and Y coordinates of the start and end are equal and greater than 1 (i.e. a diagonal). Unfortunately, this isn’t pretty to look at. To correct this we have to reduce our diagonal by one row and one column and introduce a curve like this:
<li data-coords="2,2"><a href="http://jqueryui.com/demos/accordion/">Accordion</a></li> <li data-coords="4,2"><a href="http://jqueryui.com/demos/autocomplete/">Autocomplete</a></li> <li data-coords="5,3" data-dir="E"></li> <li data-coords="6,4" data-dir="S"></li> <li data-coords="7,4"></li> <li data-coords="8,3" data-dir="E"></li> <li data-coords="8,2"></li>
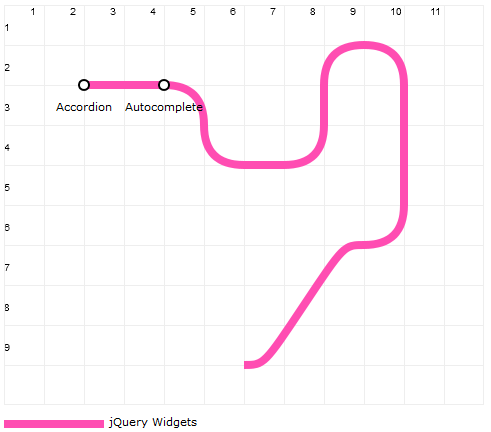
And there we have it, nice curves again and no sharp turns. Let’s wrap-up this line (no pun intended) and move on to multiple lines, markers and labels. I have now updated the markup to extend the first line a little and then added a second line shown in green labeled “jQuery Interactions.”
<div data-columns="12" data-rows="10" data-cellsize="40" data-legendid="legend" data-textclass="text" data-gridnumbers="true" data-grid="true" data-linewidth="8"> <ul data-color="#ff4db2" data-label="jQuery Widgets"> <ul data-color="#ff4db2" data-label="jQuery Widgets"><li data-coords="2,2"><a href="http://jqueryui.com/demos/accordion/">Accordion</a></li></ul> </ul> <ul data-color="#ff4db2" data-label="jQuery Widgets"> <ul data-color="#ff4db2" data-label="jQuery Widgets"><li data-coords="4,2"><a href="http://jqueryui.com/demos/autocomplete/">Autocomplete</a></li></ul> </ul> <ul data-color="#ff4db2" data-label="jQuery Widgets"> <ul data-color="#ff4db2" data-label="jQuery Widgets"><li data-coords="5,3" data-dir="E"></li></ul> </ul> <ul data-color="#ff4db2" data-label="jQuery Widgets"> <ul data-color="#ff4db2" data-label="jQuery Widgets"><li data-coords="6,4" data-dir="S"></li></ul> </ul> <ul data-color="#ff4db2" data-label="jQuery Widgets"> <ul data-color="#ff4db2" data-label="jQuery Widgets"><li data-coords="7,4"></li></ul> </ul> <ul data-color="#ff4db2" data-label="jQuery Widgets"> <ul data-color="#ff4db2" data-label="jQuery Widgets"><li data-coords="8,3" data-dir="E"></li></ul> </ul> <ul data-color="#ff4db2" data-label="jQuery Widgets"><li data-coords="8,2"></li></ul> </div>
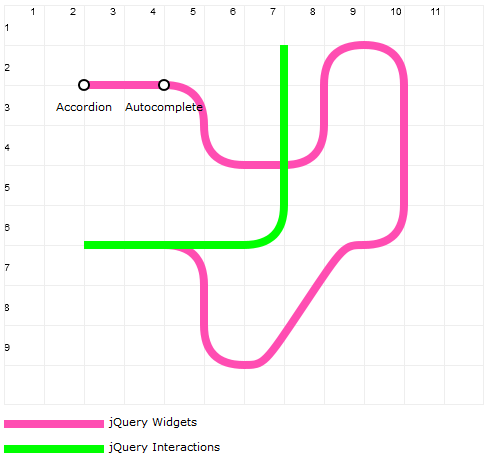
In this map, there is a portion where both lines overlap. This is a fairly common situation and if you do nothing, the line that is drawn last will be at the top. This is less than ideal for communicating information, let alone train routes. To solve this problem, the plugin allows you to “shift” a line in X and/or Y directions by a multiple of the chosen line thickness.
(UL) data-shiftCoords: The number of line-widths by which line should be shifted in any direction specified as an X,Y pair with negative values indicating shift closer to the origin (0,0). You could manually do the shift by specifying precise coordinates, however this can make the line more complicated to define. (Example: data-shiftCoords=”-1,0″ means move the line to the left by 1 line width.)
<ul data-color="#00ff00" data-label="jQuery Interactions" data-shiftCoords="0,-1">
With the above change, our map now looks like this:
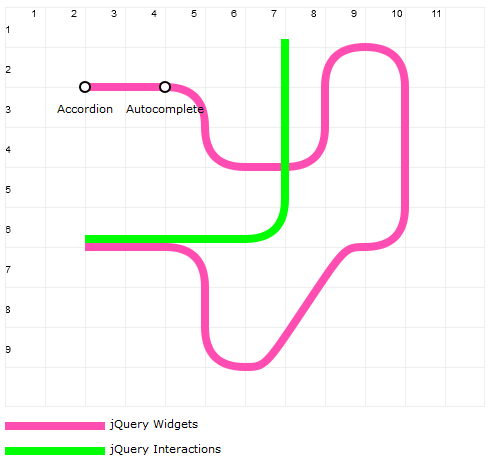
Much nicer and simpler since we did not have to make any changes to the individual line coordinates. Next, let’s add some markers. The plugin supports three types of markers: stations, interchanges and extended interchanges. These are always black-and-white (or white-and-black) and can be placed anywhere on a line. The attributes for markers are:
(UL) data-reverseMarkers: If the markers should be rendered white on black instead of the default, black on white. The default is “false”.
(LI) data-marker: Can be either “station” or “interchange.” Will produce a different marker for each. Value may be prefixed by “@” to indicate that the LI element is solely for indicating the position of the marker and should not be used as a coordinate defining the path of the line. (Examples: data-marker=”station” or data-marker=”@interchange”)
IMPORTANT: Markers are displayed only if the LI element contains content. Interchange markers ignore any coordinate shift values specified for the line.
(LI) data-markerInfo: For “interchange” or “@interchange” markers, this attribute is used to define scenarios in which the interchange marker has to “stretch” across multiple lines or connect lines that are not next to each other. The attribute value consists of a letter “v” for vertical or “h” for horizontal, followed by a number representing the number of line widths to stretch (example: v3 or h4). The marker is rendered at the coordinate position specified and extends either vertically upwards or horizontally to the right.
While it is easy and convenient to use the LI elements that define line coordinates to also define markers, sometimes this does not yield the best result. The marker may appear off by a few pixels. In these cases, it is best to add an LI element for the marker, use the “@” prefix and provide precise coordinates with decimal places (Example: 1.5,2.25).
Here is the markup and map again, updated with station and interchange markers.
<div data-columns="12" data-rows="10" data-cellsize="40" data-legendid="legend" data-textclass="text" data-gridnumbers="true" data-grid="false" data-linewidth="8"> <ul data-color="#ff4db2" data-label="jQuery Widgets"> <ul data-color="#ff4db2" data-label="jQuery Widgets"> <li data-coords="2,2" data-marker="interchange"><a href="http://jqueryui.com/demos/accordion/">Accordion</a></li> <li data-coords="4,2"><a href="http://jqueryui.com/demos/autocomplete/">X</a></li> <li data-coords="5,3" data-dir="E"></li> <li data-coords="5,7" data-marker="@station">X</li> </ul> </ul> <!-- marker-only node --> <ul data-color="#ff4db2" data-label="jQuery Widgets"> <ul data-color="#ff4db2" data-label="jQuery Widgets"> <li data-coords="6,4" data-dir="S" data-marker="interchange" data-markerinfo="h5">X</li> <li data-coords="7,4"></li> <li data-coords="7.15,8" data-marker="@station">X</li> </ul> </ul> <!-- marker-only node, moved to the right by 0.15 --> <ul data-color="#ff4db2" data-label="jQuery Widgets"> <li data-coords="8,3" data-dir="E"></li> <li data-coords="8,2"></li> <li data-coords="9,1" data-dir="N"></li> <li data-coords="10,2" data-dir="E" data-marker="interchange">X</li> <li data-coords="10,5"></li> <li data-coords="9,6" data-dir="S" data-marker="station">X</li> <li data-coords="6,9"></li> <li data-coords="5,8" data-dir="W"></li> <li data-coords="5,7"></li> <li data-coords="4,6" data-dir="N"></li> <li data-coords="2,6">Tabs</li> </ul> <ul data-color="#00ff00" data-label="jQuery Interactions" data-shiftCoords="0,-1"> <!-- marker-only node, moved up by 0.10 --> <ul> <li data-coords="5,6" data-marker="@station">X</li> <li data-coords="6,6"></li> <li data-coords="7,3" data-marker="@station">X</li> <li data-coords="7,5" data-dir="E" data-marker="station">X</li> <li data-coords="7,1" data-marker="interchange">X</li> </ul> </div> <div id="legend"></div>
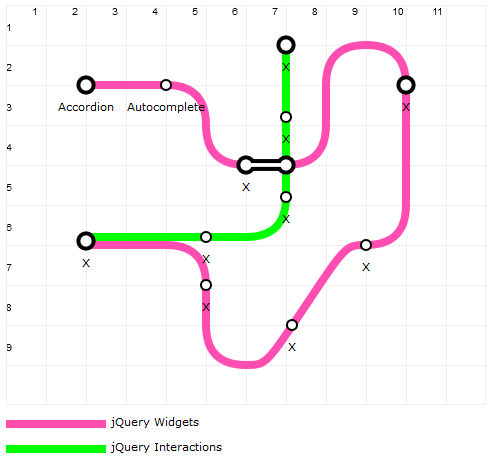
I used the label “X” for all the station or interchange names. The only thing left to do is change the labels and also position them so they don’t appear on top of the line. The attribute used for positioning the labels is:
(LI) data-labelPos: Specifies where the label should be displayed using a directional abbreviation. Supported values are N, E, S, W, NE, NW, SE, SW. (Default is “S”). Sometimes your label may be too long and text-wrap may be needed. To do this, you can use “\n” within the text of the label (<br />will not work since the only markup supported for a label is the <a> element).
Labels are added as absolutely positioned <span> elements and when the map is added inside a DOM element with a complex, CSS layout, they may not always appear in the right place.
Let’s set the label text and position, turn off the grid and look at the final markup and rendered map.
<div data-columns="12" data-rows="10" data-cellsize="40" data-legendid="legend" data-textclass="text" data-gridnumbers="true" data-grid="false" data-linewidth="8"> <ul data-color="#ff4db2" data-label="jQuery Widgets"> <ul data-color="#ff4db2" data-label="jQuery Widgets"> <li data-coords="2,2" data-marker="interchange"><a href="http://jqueryui.com/demos/accordion/">Accordion</a></li> <li data-coords="4,2"><a href="http://jqueryui.com/demos/autocomplete/">Auto\ncomplete</a></li> <li data-coords="5,3" data-dir="E"></li> <li data-coords="5,7" data-marker="@station" data-labelpos="W">Slider</li> </ul> </ul> <!-- marker-only node --> <ul data-color="#ff4db2" data-label="jQuery Widgets"> <ul data-color="#ff4db2" data-label="jQuery Widgets"> <li data-coords="6,4" data-dir="S" data-marker="interchange" data-markerinfo="h5">Date\npicker</li> <li data-coords="7,4"></li> <li data-coords="7.15,8" data-marker="@station" data-labelpos="E">Dialog</li> </ul> </ul> <!-- marker-only node, moved to the right by 0.15 --> <ul data-color="#ff4db2" data-label="jQuery Widgets"> <li data-coords="8,3" data-dir="E"></li> <li data-coords="8,2"></li> <li data-coords="9,1" data-dir="N"></li> <li data-coords="10,2" data-dir="E" data-marker="interchange" data-labelpos="E">Button</li> <li data-coords="10,5"></li> <li data-coords="9,6" data-dir="S" data-marker="station">Progress\nbar</li> <li data-coords="6,9"></li> <li data-coords="5,8" data-dir="W"></li> <li data-coords="5,7"></li> <li data-coords="4,6" data-dir="N"></li> <li data-coords="2,6">Tabs</li> </ul> <ul data-color="#00ff00" data-label="jQuery Interactions" data-shiftCoords="0,-1"> <!-- marker-only node, moved up by 0.10 --> <ul> <li data-coords="5,6" data-marker="@station" data-labelpos="N">Selectable</li> <li data-coords="6,6"></li> <li data-coords="7,3" data-marker="@station" data-labelpos="W">Resizeable</li> <li data-coords="7,5" data-dir="E" data-marker="station" data-labelpos="E">Droppable</li> <li data-coords="7,1" data-marker="interchange" data-labelpos="W">Draggable</li> </ul> </div> <div id="legend"></div>
There you have it…a map that faithfully reproduces the style of the London Underground map, but hopefully, is not too difficult for you to create once you get familiar with the custom attributes required in the HTML markup to render the map. Enjoy!
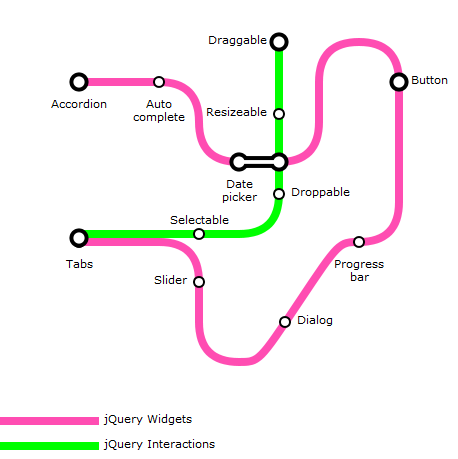
Reference
(DIV) data-columns: The number of columns the map will display (default=10)
(DIV) data-rows: The number of rows the map will display (default=10)
(DIV) data-cellSize: The width and height of each cell in pixels (default=100)
(DIV) data-legendId: The ID of an HTML element into which the map legend will be appended
(DIV) data-textClass: The CSS class to use for text labels in the map
(DIV) data-grid: True or false, to show or hide a grid that is useful during map construction (default=false)
(DIV) data-gridNumbers: True or false, to show or hide numbers on the grid. Only applies if data-grid=”true” (default=true)
(DIV) data-lineWidth: The width in pixels for each line (default=10)
(UL) data-color: The color of the line in standard CSS RGB notation
(UL) data-label: The label for the line that will be displayed in the legend
(UL) data-shiftCoords: The number of line-widths by which line should be shifted in any direction specified as an X,Y pair with negative values indicating shift closer to the origin (default=0,0)
(UL) data-reverseMarkers: If the markers should be rendered white on black instead of the default, black on white (default=false)
(LI) data-coords: The X,Y coordinate pair where the line should be drawn to from its last location (or the starting location if it’s the first LI element)
(LI) data-marker: Can be either “station” or “interchange.” Will produce a different marker for each. Value may be prefixed by “@” to indicate that the LI element is solely for indicating the position of the marker and should not be used as a coordinate defining the path of the line
(LI) data-markerInfo: For “interchange” or “@interchange” markers, this attribute is used to define scenarios in which the interchange marker has to “stretch” across multiple lines or connect lines that are not next to each other. The attribute value consists of a letter “v” for vertical or “h” for horizontal, followed by a number representing the number of line widths to stretch (example: v3 or h4). The marker is rendered at the coordinate position specified and extends either vertically upwards or horizontally to the right.
(LI) data-labelPos: Specifies where the label should be displayed using a directional abbreviation. Supported values are N, E, S, W, NE, NW, SE, SW (default=S)
Dev
great tool
Johannes Ellenberg
WoW! You rock! Thanks for sharing :)
Geert
the link to the demo goes to an empty page
Narendra Vaghela
excellent tool
Dave
Does this script downgrade for folks without javascript loaded? Also, I was wondering if you've found any cross-browser issues. Thanks!
Alfonso Rivero
great
Demo
so cool
wonderman
A great tool with great passion. Excellent! However, I tried the plugin and found that it removed any extra attributes that I added. For example, I would like to refer to a inside- , so I added an id property to the . After the map was generated, the id propertied had been removed. Do you have a workaround on this?
nik
@wonderman The plugin swaps out the unordered list and replaces it with a canvas element, so there is no way to refer to any of the original elements once the script has created the subway map. If you need to do something with the <li> elements, I suggest you do it before the plugin renders as the DOM is irreversibly changed once the rendering is done.
Daniel Doctor
Hi, It's a super cool library. By the way we are using it on http://www.metro.smartdsign.net/ with a route trace (minimal distance between stations), Hope you like it!
Renyai_renyai
its damn cool man.....nice plugin...
Francesco
Talking about you on RetroBottega!!! http://www.retro-bottega.com/2011/04/23/the-inspector-02-subway-map-plugin/
Simon
I definitely like the end result, but adding all your custom attributes (such as "data-color") to the div, ul and li elements produces invalid HTML code. For many people this may not be an issue, but it should be possible to create valid pages with every plugin. Therefore I would propose that all definitions should be moved out of HTML into an independent XML file, which is actually the best-practise solution for defining data. Then you could do something like this: [HTML]: [XML]: 12 [..] #ff4db2 [..] <segment label="Accordion"> 2,2 [..] [JS]: $.get('map.xml', function(xml) { // work with the already-parsed XML var lines = $('line', xml); [..] var segments = $('segment', $(lines[0]))W [..] }); This would turn your awesome solution into a fabulous solution :-). Let me know what you think
techbubble
@Simon: I did a lot of research and was careful to ensure that the HTML markup was valid. This is why I chose the data-* approach, which is the recommended way to add custom attributes per the (evolving) HTML 5 spec. Even jQuery supports these attributes natively. http://www.google.com/search?aq=f&sourceid=chrome&ie=UTF-8&q=html5+data-* As far as moving it to a separate file, while it would work in many situations where you have unfettered access to the server to create files, it would not work in scenarios where you just want to embed some HTML on a page and be done. I think the XML approach could be in addition to the attribute approach, instead of replacing it. I will include it in the next (overdue) release. Thanks for the feedback and tip.
Jiří Pudil
The data attribute name (prefixed with data-) should not contain uppercase letters though, see http://html5doctor.com/html5-custom-data-attributes/
Béla Varga
Hi, really nice work! But can you put it to github so that i can fork it and can add more features. thx
Béla Varga
Hi, really nice work! But can u add it please to github so that i can fork it. thx
Useful JavaScript Libraries and jQuery Plugins Part 1 « OhMyDev
[...] [...]
The most widely used 32 jQuery plugin demo Web Page Design | Web Page Design Templates | Html5 Template
[...] Subway Map Visualization jQuery Plugin [...]
21 of the most commonly used jQuery plugin demo and download Web Page Design | Web Page Design Templates | Html5 Template
[...] Subway Map Visualization jQuery Plugin [...]
30 Excellent jQuery Plugins for Navigation and Menus
[...] → More Info [...]
w3easystep » Blog Archive » 30 Fantastic New jQuery Plugins
[...] Subway Map Visualization Plugin The amazing Subway Map Visualization plugin allows you create, interactive subway map visualizations with HTML. Its style has been based on Londons Underground maps, with smooth curves & interchange connectors and 45-degree diagonals. The map size, line width and colors can all be customizable and the markup is also search engine friendly. Subway Map Visualization PluginDemo [...]
35+ Useful jQuery Menu Plugins « Next Step...Innovate
[...] [...]
My 2012 web design and marketing round-up of all things awesome.
[...] [...]
Matt
fix for IE is changing line 118 from self=this; to var self=this; see IE on inner / outer window and self issues / global variable scope.
Colección de librerías JavaScript y jQuery, tercera parte: mejora de la productividad, mapas, gráficos e imágenes.
[...] Subway Map Visualization jQuery Plugin [...]
DevCast Weekly #2 | DevCast
[...] 58:00Subway uma lib só para você construir mapas de metro. [...]
DevCast Weekly #2 | João Vagner
[...] Subway uma lib só para você construir mapas de [...]
Camilo
This is awesome! Is there a way to split or fork a line? Say, I have one station that leads to two different stations that are not linear, they are rather parallel one another, but belong to the same line… Nested UL's perhaps?
32个极其有用的jQuery插件! | 有日历
[...] Subway Map Visualization jQuery Plugin [...]
Reza R
Very helpful library thanks. 1- I would like to display a popup message when a user click on station, could you help me how ? 2- Is there zooming possibility ? I would like to scale the metro map to mobile phone screen and I am looking for a zoom feature.
Phil
Is it possible to convert this to render an SVG? - this would then make the individual stations interactive. We could then listen for events on each station.
giordy
Hi, is there a way to render canvases using relative position, reather then absolute? So label positions can resize if page resizes
35+ Useful jQuery Menu Plugins | Script Hunter
[...] [...]
Diego
Awesome plug-in. Once you get the hang of it, it becomes very easy to customize. Many, many thanks!
Eric
Very cool! Great work. Fun to play with. Thanks for sharing.
Useful JavaScript Libraries and jQuery Plugins For Web Developers
[...] Maps, Graphs:world maps – subway map – Google maps – open source maps – SVG fallback – gauges – graphs [...]
20 Examples of Inspiring jQuery Maps Plugins | Orphicpixel
[...] Subway Map Visualization [...]
85 Best jQuery Plugins in 2011Wordpress Developent Websoite
[...] Subway Map Visualization jQuery Plugin [...]
ochien
this if fucking crazy plugin.....awesome !!! thx to create this :)
Drawing MBTA Commuter Rail Map using their CSV and D3 | TechSlides
[...] Subway Map Visualization jQuery Plugin D3 Click Zooming in JSfiddle D3 Drawing lines based on points and moving a circle on arcs Subways of North America Image on XKCD Meteor and D3 to generate SVG CSS Tube Map – London Underground map with just CSS Paris Subway Map with D3.js and SVG [...]
Markus
While playing around with the plug-in, and following the step-by-step implementation tutorial, I found an issue with label positioning. The label's positions are calculated in dependency to the position of the $("sampleselector") relative to the webpage's origin. To fix that, you have to use plain positions in the label markup code in line 405:
var style = (textClass != "" ? "class='" + textClass + "' " : "") + "style='" + (textClass == "" ? "font-size:8pt;font-family:Verdana,Arial,Helvetica,Sans Serif;text-decoration:none;" : "") + "width:100px;" + (pos != "" ? pos : "") + ";position:absolute;top:" + (y) + "px;left:" + (x) + "px;z-index:3000;'";
Then add the following line before "self = this;" in line 123.el.css("position", "relative");
This way the labels are positioned relatively to the parent div. Also, I think it's handy to remove the comment slashes in lines 121 and 122, so the element will have the size of the map's canvas and following page content won't be rendered below the map.16 Best Free jQuery Map Plugins | EagleTuts
[...] 11. Subway Map [...]
10+ Best Free jQuery Map Plugins | Daily Creative Design
[...] 4. Subway Map [...]
Html Css Jquery Javascript Based Super Powerful jQuery Navigation Menus and Menu Plugins Tutorials | Thenewsblock
[…] 58. Subway Map Visualization jQuery Plugin […]
20+ jQuery Grafik Eklentileri
[…] Detail […]
Useful JavaScript Libraries and jQuery Plugins For Web Developers
[…] Maps, Graphs:world maps – subway map – Google maps – open source maps – SVG fallback – gauges – graphs […]
Giovanni
Hello Nik, congratulations for the amazing plugin subwayMap! A resource commendable! I would like to implement the plugin in my website www.giovanniscafaro.it but do not know how to install it. I know the basics of HTML and CSS, but I do not know what should I do to install this wonderful plugin in my wordpress. It would be nice if you would do for us newbies a step by step guide so that we can install subwayMap wordpress sites, so that we can access this wonderful resource and run it in the site? Then with a little practice we will definitely work. Thank you again. John, Naples, Italy.
Milan sompura
I have used this chart into my application but I can't show the lines above the 100. it does not take more than 100 parallel lines into one graph. what should i do to resolve this issue
Milan sompura
Page unresponsive error occurred when continuously access the page if data is more than 100 parallel lines. its also too slow if there is more data available.
Useful JavaScript Libraries and jQuery Plugins | Smashing Magazine
[…] Maps, Graphs:world maps42 – subway map43 – Google maps44 – open source maps45 – SVG fallback46 – gauges47 – […]
Feather
IE8 can't be displayed properly. The version is 8.0.7601.17514
Web Design Software
[…] Subway Map Visualization […]
Brendan
I really loved this tutorial. I was able to create the railroad map I wanted with relative ease however I am now trying to add it into a rail/angular application and I'm having a hard time. Is there a best way to get this to work with rails?
Deyang Kong
very very very very very great . think you. that i use her to show pipeline.
Richard Taylor
Incredible plugin! Thank you so much! I used it to help one of our customer visualise their customer journey and interactions with different staff and departments and it really helped us figure out where the processes could be improved upon! Wonderful stuff!
N.Dinesh
Hi great work man. i need some click event in this map. can you possible to uploaded click event code ?
Rajini
We need to add a click event inside the map. Is it possible ?
Rushabh
Amazing plugin. Really appreciate the work you have put into it. I had a question - Could we add elements to the tags using DOM and then replot the subway map without relaoding the page somehow? I tried doing that but the plugin seems to be reading the source file only and does not consider the dynamic elements that i have added using DOM
Custom Subway Maps for Digital Signage
[…] week we have a real treat for you – a Subway Map Visualization jQuery Plugin. What the heck does that mean? It means that you can build your own custom maps and use them in […]
gema
can this plugin use jquery tooltip when hover the node? would you like to give me an example? Thanks :)
Create Interactive Subway Map with jQuery Plugin - Azher Ahmed
[…] Subway Map Visualization jQuery Plugin lets you create a beautiful, interactive subway map visualization for their website using HTML markup. The map size, line width and colors can all be customizable. The markup used to create the map is search engine friendly too. […]
ToddW
Any issues with device compatibility? ...with responsive design?
Bob
Great work, does the job brlliantly. Just one question, I am using it as a Navigation tool. Is there a way in which I can have a dot in the middle of circle to indicate what "station/page" I am on?
FormByFunction
Thank you for the solution of @Markus to make this plugin responsive, but the y position is still a bit off, with the following change, it works perfectly for me:
var style = (textClass != "" ? "class='" + textClass + "' " : "") + "style='" + (textClass == "" ? "font-size:8pt;font-family:Verdana,Arial,Helvetica,Sans Serif;text-decoration:none;" : "") + "width:100px;" + (pos != "" ? pos : "") + ";position:absolute;top:" + (y - (topOffset > 0 ? topOffset : 0)) + "px;left:" + (x) + "px;z-index:3000;'";
bFacio
What a nice plugIn... I tested in a very simple demo and it works as easy as is. But now I have a doubt'bout how to fill with diferent colors the nodes marked as points. Do you have an example of how to do this? Best regards.
Kartik Online | Visualizing workflows
[…] I looked around for subway map generators and found one that Nik Kayani implemented using jQuery a while ago. Nik’s original post is here. […]
35+ Useful jQuery Menu Plugins – Help Users Navigate Better!
[…] […]
Subway Map Visualization jQuery Plugin - Pixel Library
[…] Learn Extra Demo […]
Paul Hinrichsen
A HUGE THANKS for a BRILLIANT Tool. I have spent MONTHS searching for a means of creating the London Underground effect for a map which I am working on. Is it possible to choose a set of pairs of coordinates and then to draw an Arc joining them rather than joining each successive pair in the group with a straight line. Thanks Paul
waqas
How can I add custom shapes (Point, Polygon. etc) on different places of this map?
phani
I am trying to use this jquery with Spring MVC, i am not able to render the connection lines, can some one help me out.
PSD Junction
Amazing and Much Useful Post.
Tom
I tried the last code snippet for the complete sample which isn't rendered in my browser as shown here in the screenshot. The snippet contains nested ul-elements and lots of elements all labeled with "jQuery Widgets", why are there different elements?
Tom
And it seems to me "data-shiftCoords" does not work properly for data-marker="interchange"...?
google1,
Do you mind if I quote a few of your posts as long as I provide credit and sources back to your site? My blog site is in the very same area of interest as yours and my visitors would genuinely benefit from some of the information you provide here. Please let me know if this ok with you. Cheers!